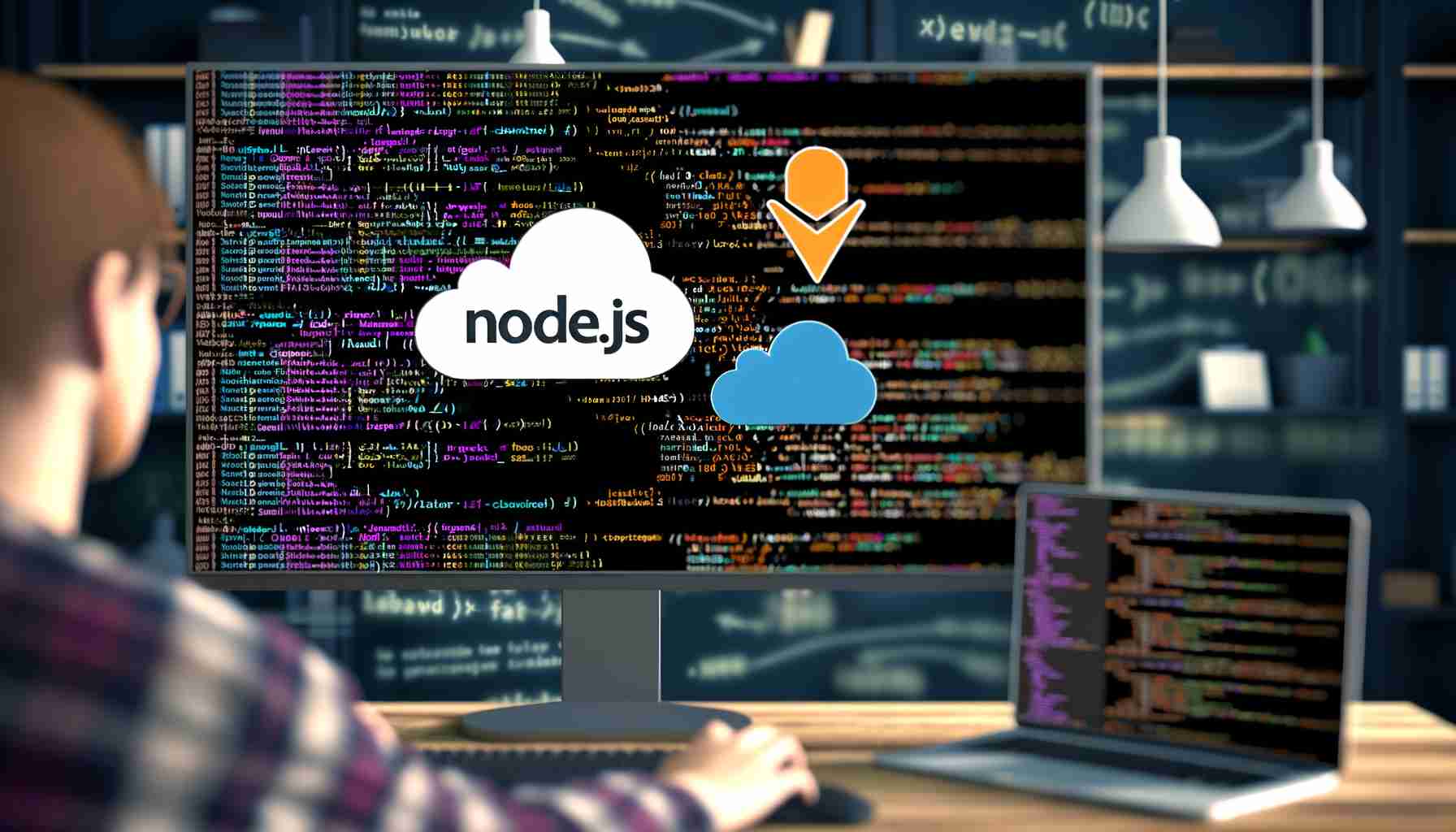
Understanding Node.js and AWS Lambda
Understanding Node.js and AWS Lambda
Node.js is a runtime environment that allows JavaScript to be executed on the server side. It uses an event-driven, non-blocking I/O model. This makes it lightweight and efficient. A perfect match for building fast, scalable applications that need to handle numerous requests simultaneously. AWS Lambda is a serverless computing service. It runs your code in response to events without provisioning or managing servers. This means you can focus on writing your application without worrying about infrastructure. When you combine Node.js with AWS Lambda, you get the best of both worlds. The asynchronous capabilities of Node.js shine in a serverless environment. You can execute code in parallel without waiting for other processes to finish. This is ideal for microservices architectures.- Asynchronous Nature: Node.js handles multiple operations at once. It doesn’t freeze the execution while waiting for I/O operations to complete.
- Scalability: AWS Lambda automatically scales your applications by running code in response to events. You can handle any traffic volume without manual intervention.
- Cost-Effectiveness: With AWS Lambda, you only pay for what you use. This model is ideal for applications with variable workloads.
- Easy Deployment: Node.js functions can be easily deployed on AWS Lambda. You can use the AWS CLI, SDK, or the AWS Management Console for deployment.
exports.handler = async (event) => {
const response = {
statusCode: 200,
body: JSON.stringify('Hello from Lambda!'),
};
return response;
};
In your Lambda console, select Node.js as your runtime. Upload your code, and it’s ready to go. A
notable part of Node.js development is using its SDK for JavaScript. It simplifies interaction with
AWS services. You can install it with npm:
npm install aws-sdk
With the SDK, you can easily access services like S3, DynamoDB, and others. This powerful
combination allows you to build complex applications with minimal overhead. The synergy between
Node.js and AWS Lambda positions developers to create robust, serverless applications. You focus on
application logic. The platform takes care of the scaling. For more on serverless architectures,
check out this article on
serverless architecture.Setting Up Your Environment
Creating a Node.js environment for developing workloads in the cloud requires attention to detail. Below are the essential steps to achieve this. First, install Node.js and npm. They are pivotal for your development. Use the package manager relevant to your operating system. For Ubuntu, run:sudo apt update
sudo apt install nodejs npm
For Mac, use Homebrew:
brew install node
For Windows, download the installer from the official website. Next, ensure you have AWS CLI
installed. This tool allows you to manage AWS services directly from your terminal. Installation
steps for the AWS CLI may differ by platform but aim for the latest version. On Ubuntu, for example,
you can execute:
sudo apt install awscli
You’ll need to configure your AWS credentials next. Execute the following command in your terminal:
aws configure
This will prompt you for four details:
- AWS Access Key ID
- AWS Secret Access Key
- Default region name
- Default output format
npm install aws-sdk
This installs the SDK as a dependency for your project. Post-installation, include it at the start
of your Node.js files:
const AWS = require('aws-sdk');
You can now access multiple AWS services such as S3, DynamoDB, and Lambda. Make use of the SDK’s
promise-based methods. This approach allows for cleaner asynchronous operations. For example,
uploading files to an S3 bucket becomes simplified:
const s3 = new AWS.S3();
const params = {
Bucket: 'your-bucket-name',
Key: 'file.txt',
Body: 'Hello World'
};
s3.upload(params).promise()
.then(data => console.log('Upload Success', data))
.catch(err => console.log('Upload Error', err));
For more details on the AWS SDK for JavaScript, check this
beginner's guide. This
environment setup lays a solid foundation for your Node.js development on the cloud.Best Practices for Writing Node.js Lambda Functions
Writing Node.js functions for AWS Lambda can feel like walking a tightrope. It's all about balance: simplicity, performance, and reliability. Here are some vital practices to enhance your coding experience and outputs. Keeping your functions succinct is paramount. A function should ideally do one thing and do it well. When the complexity creeps in, your function becomes harder to read and maintain. This simplicity enables faster debugging and fewer chances of error. Error handling requires thoughtful consideration. Utilize try-catch blocks to gracefully manage exceptions. Be explicit about the errors you catch and respond accordingly. Here's a simple example:
const handler = async (event) => {
try {
// Your main logic here
} catch (error) {
console.error("An error occurred:", error);
throw new Error("Internal Server Error");
}
};
Always log errors to a monitoring service for visibility. This can save you headaches down the road.
Integrate this with alerts to notify you of issues. Optimizing package size is incredibly important.
Unused dependencies bloat your function. Use tools like tree-shake or analyze tools to keep your
package lean. Aim for a package size under 5 MB to minimize cold start times. Using
async/await is essential for managing asynchronous code. It makes your code cleaner and
easier to understand. Avoid callback hell at all costs! Here's how you can implement it effectively:
const handler = async (event) => {
const data = await fetchData();
return {
statusCode: 200,
body: JSON.stringify(data)
};
};
Cold start issues can be frustrating. Pre-warming strategies can help mitigate these. Schedule
invocations at regular intervals to keep your functions warm. Use the provisioned concurrency
feature for consistent performance. Take advantage of environment variables for configuration. This
keeps sensitive data out of your codebase and makes it easier to manage different environments. Use
a .env file in development to streamline this. Leverage AWS services wisely. If you hook into S3 or
DynamoDB, ensure that your connections are reused rather than created anew each time. This improves
performance and resource utilization. For more on efficient Lambda function integration with AWS
services, check out the detailed guide
here.Integrating with AWS Services
Integrating Node.js Lambda functions with AWS services maximizes performance and functionality. Here, we’ll navigate some best practices and real-world scenarios that highlight effective integrations. Start with DynamoDB, a popular choice for serverless applications. Use the AWS SDK to make interactions seamless. When fetching or saving data, utilize promises for clean, manageable code. For example, consider a function that stores user profiles:
const AWS = require('aws-sdk');
const dynamoDB = new AWS.DynamoDB.DocumentClient();
exports.handler = async (event) => {
const params = {
TableName: 'Users',
Item: {
userId: event.userId,
name: event.name,
email: event.email,
},
};
try {
await dynamoDB.put(params).promise();
return { statusCode: 200, body: 'User profile created.' };
} catch (error) {
return { statusCode: 500, body: error.message };
}
};
Make sure to handle errors gracefully. Implement retry logic to enhance reliability. DynamoDB's
built-in exponential backoff can work wonders here. Next, let’s pivot to S3, the go-to choice for
storing files. Integrate S3 with Lambda for handling uploads, downloads, or image processing. For
uploads, generate pre-signed URLs to allow client-side uploads without exposing your credentials.
Here’s a sample function for generating a pre-signed URL:
const s3 = new AWS.S3();
exports.handler = async (event) => {
const bucketName = 'your-bucket-name';
const fileName = event.fileName;
const params = {
Bucket: bucketName,
Key: fileName,
Expires: 60, // URL expiry time in seconds
};
try {
const url = await s3.getSignedUrlPromise('putObject', params);
return { statusCode: 200, body: url };
} catch (error) {
return { statusCode: 500, body: error.message };
}
};
Integrating with API Gateway allows you to expose your Lambda functions as RESTful APIs. Define
routes that map to different Lambda functions, and configure authentication and caching. Remember to
manage CORS settings when exposing APIs. Ensure every response includes the right headers to
facilitate cross-origin requests. Adjust Lambda function timeout settings to accommodate
longer-running processes. For example, image processing might take longer than your usual function.
Set timeouts wisely to avoid unnecessary failures. Incorporate monitoring and logging solutions to
track Lambda performance. Use structured logging for easier debugging and analysis. CloudWatch
provides robust options for tracking API calls and resource usage. Consider checking out this
guide on logging in Lambda.
It’ll help elevate your monitoring game, ensuring operational excellence while seamlessly
integrating with AWS services.Monitoring and Logging
Monitoring and logging are crucial in a Node.js AWS Lambda environment. They enable you to grasp application performance and detect issues effectively. A robust monitoring and logging framework can enlighten the darkness of development and operations.Start with CloudWatch for your monitoring needs. It's deeply integrated with AWS services and straightforward to set up. You can track metrics such as duration, invocations, and error rates. Setting up alarms based on these metrics can notify you about any abnormalities rapidly. This way, you keep your environment healthy without constantly checking it.
Yet, while CloudWatch is great, it can become pricey as your logs overflow. Open-source solutions are emerging to alleviate costs while providing flexibility. Tools like Grafana with Loki can help you visualize log data. They also offer a different perspective on performance trends, which is invaluable.
When logging events in your Lambda functions, it’s essential to structure your logs. Adopting a consistent format aids in quickly filtering and analyzing logs. For instance, you might log user actions and system events differently. Consider using JSON for structured logging:
const logEvent = (event) => {
console.log(JSON.stringify({
timestamp: new Date().toISOString(),
event: event,
}));
};
Also, make extensive use of log levels. Use info for operational events, warn for potential issues, and error for failures. This approach helps keep logs manageable and grants better insights into what’s happening in production.
Regularly reviewing your logs is essential. Set up a schedule to delve into your log data, seeking trends or anomalies. This process allows for proactive problem resolution, preventing potential downtime.
In addition to these methods, consider utilizing tracing solutions. Implementing distributed tracing enables you to see requests traveling through various services. This visibility allows you to pinpoint bottlenecks and enhance performance.
Finally, your monitoring and logging strategy must accommodate scaling. As your application grows, both your logging volume and monitoring requirements will expand. Designing your logging to be efficient while ensuring your monitoring remains capable is necessary for operational excellence. To explore more on monitoring trends, check this resource for setting up Grafana with Loki.